Custom Message Parsers
When learning to create custom parsers for LogViewPlus it is helpful to begin with the sample code projects. This tutorial will assume that you have downloaded and run the sample projects successfully. Please see running the samples for more information.
Custom message parsers are helpful when want to extract data from a log entry message in a way that is not supported by the standard Message Parser configuration. Creating custom message parsers is probably the most advanced LogViewPlus extension type because you must correctly implement three interfaces:
1. ILogParser - This interface is described in Custom Parsers.
2. ILogParserWithCustomMessageTemplates - Enhances your ILogParser implementation to return a ICustomMessageTemplate.
3. ICustomMessageTemplate - The implementation for your custom message parser.
Our custom parser implementation will be responsible for parsing a very simple log file which will contain XML log entries. The custom message parser will analyze the attributes in the XML selectively to determine possible extracted values.
If we look at the code included in the CustomParser project, there is a class called CustomXmlParserWithCustomTemplates. and remove of the comments, we can see the custom parser implementation is straightforward.
public class CustomXmlParserWithCustomTemplates : CustomXmlParser, ILogParserWithCustomMessageTemplates
{
public int MessageTemplateFormat => 0;
public string MessageTemplateInputPrompt => "Please supply a list of attribute names.";
public ICustomMessageTemplate CreateMessageTemplate(LogEntry entry, string messageTemplate, out string errorMessage)
{
errorMessage = null;
if (string.IsNullOrEmpty(messageTemplate))
{
errorMessage = "Message template cannot be null.";
return null;
}
var template = TemplateFromString(messageTemplate);
using (var reader = new XmlTextReader(new StringReader(entry.OriginalLogEntry)))
{
reader.Namespaces = false;
reader.ReadToFollowing("MESSAGE"); // Initialize read to get to the target attributes.
reader.MoveToNextAttribute(); // Timestamp is always the first attribute. Not needed here.
var attributes = GetAttributes(reader);
return MyCustomMessageTemplate.Create(template, attributes, entry.Message);
}
}
public void SetCustomMessageTemplates(string[] templates)
{
_savedMessageTemplates.Clear();
if (templates == null)
return;
foreach (var template in templates)
{
var set = TemplateFromString(template);
_savedMessageTemplates.Add(set); // Save all received templates for future use.
}
}
}
Note that CustomXmlParserWithCustomTemplates is a sample implementation of the ILogParserWithCustomMessageTemplates interface:
public interface ILogParserWithCustomMessageTemplates
{
int MessageTemplateFormat { get; }
string MessageTemplateInputPrompt { get; }
void SetCustomMessageTemplates(string[] templates);
ICustomMessageTemplate CreateMessageTemplate(LogEntry logMessage,
string messageTemplate, out string errorMessage);
}
This interface has four parts:
MessageTemplateFormat - used to control syntax highlighting when providing a custom message template implementation. One of three values will be expected: 0 = Text, 1 = XML, 2 = JSON. All other values will result in default syntax highlighting.
MessageTemplateInputPrompt - The user prompt to be shown on the Custom Parse Message Filter dialog.
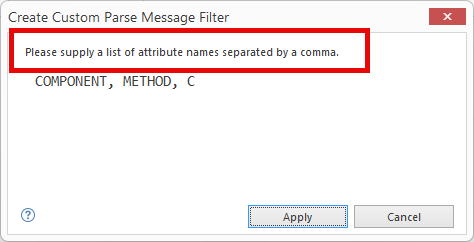
SetCustomMessageTemplates - called during parser initialization to provide the configured message templates. Only configured message templates will be provided. Default implementations are expected to be known internally by the parser implementation..
CreateMessageTemplate - used by the Custom Parse Message Filter dialog to validate and create new ICustomMessageTemplate implementations.
ICustomMessageTemplate implementations are used to represent custom message templates in LogViewPlus. Message templates consist of a series of key value pairs which are used to display information in the grid. In addition to this, the message template is also responsible for providing LogViewPlus with the log entry message. Using this approach, the log entry message can either be cached or generated on demand.
The ICustomMessageTemplate interface is straight forward to implement. Please see MyCustomMessageTemplate in the provided sample code for more information. If you have any questions or comments please feel free to contact us.