Custom Filters
When learning to create custom filters for LogViewPlus it is helpful to begin with the sample code projects. This tutorial will assume that you have downloaded and run the sample projects successfully. Please see running the samples for more information.
Custom filters are useful when you need to filter your log file in a way that is not inherently supported by LogViewPlus.
Our custom filter implementation will be responsible for enhancing the LogViewPlus text search functionality. The existing LogViewPlus text search functionality works by matching the whole term. For example, if you search for "hello world" the search will not match "hello my world". Our custom filter implementation will work slightly differently. Any spaces in the search term will be interpreted as a separator for multiple search terms. Using this approach, "hello world" will match any string with the words "hello" and "world" anywhere in the string. Both search terms will be required.
If we look at the code included in the CustomFilter project and remove of the comments, we can see the custom filter implementation is very straightforward.
public class MyFilter : ILogFilter
{
private string[] _searchTerms = null;
public string Arguments { get; private set; }
public string Name { get { return Arguments.Replace(" ", " & "); } }
public void Initialize(string arguments)
{
Arguments = arguments;
_searchTerms = arguments.Split(' ');
}
public bool Show(LogEntry logEntry)
{
foreach(var term in _searchTerms)
{
if (logEntry.Message.IndexOf(term, StringComparison.OrdinalIgnoreCase) < 0)
return false;
}
return true;
}
}
Note that MyFilter is a sample implementation of the ILogFilter interface:
public interface ILogFilter
{
string Arguments { get; }
string Name { get; }
void Initialize(string arguments);
bool Show(LogEntry logEntry);
}
This interface has four parts:
Arguments - This getter provides access to the arguments used to initialize the filter. This is important when persisting the filter as the arguments will be needed later to reinitialize a new filter.
Name - This is the name of the filter. This name will be displayed to the user in the file management list tree. In our example filter we are replacing spaces with ampersands. This is an attempt to give the user a better understanding of how our filter works.
Initialize - A custom filter is initialized with a string that is provided by the user when creating the filter. Providing an initialization string to a custom filter is optional as some filters may not need initialization. Initialization arguments can use Argument Templates.
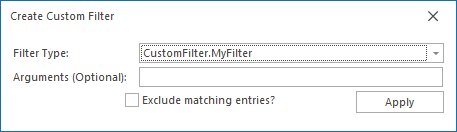
Show - The Show method is responsible for showing or hiding a given log entry. It is responsible for answering a simple question: should this log entry be displayed to the user? Custom filters may be include or exclude type filters. This functionality is implemented outside of your custom filter. The show method will always assume that your filter is written as an include filter.
As shown above, creating a custom filter for LogViewPlus is relatively simple. If you have any questions or comments please feel free to contact us.