Post Processors
When learning to create custom post processors for LogViewPlus it is helpful to begin with the sample code projects. This tutorial will assume that you have downloaded and run the sample projects successfully. Please see running the samples for more information.
The existing parsers in LogViewPlus are very powerful, but in some scenarios, you may find you want to modify the output slightly. That's where custom post processors can help. A custom post processor can be used to modify a parser's output before it is sent to LogViewPlus for display and analysis.
Let's say, for example, that we have a log file that uses custom priority levels. For example, consider the following log entries:
09:58:11,704 [75] DEBUG SyncEventService - Successfully created Event
09:58:11,704 [73] DEBUG SyncEventService - Successfully created Event
09:58:11,736 [75] Performance PerformanceWriter - Started Process entries
09:58:11,751 [81] Progress PerformanceWriter - Setting work completed
09:58:11,704 [73] DEBUG SyncEventService - Successfully created Event
Looking at these five log entries we can see three distinct log message priorities: debug, performance, and progress. The "performance" and "progress" priorities are non-standard and will not be understood by LogViewPlus. So, we are going to write custom post processor which transforms these invalid priorities into loggers which can be filtered by LogViewPlus.
Note that LogViewPlus now supports custom Log Levels. Custom log levels would be a better way to implement this functionality, but this discussion is still valid as an example.
If we look at the code included in the CustomPostProcessor project and remove the comments, we can see the custom post processor implementation is straightforward.
public class MyPostProcessor : ILogPostProcessor
{
public void Modify(LogEntry newEntry)
{
var level = newEntry.Priority;
switch (level.ToLower())
{
case "progress":
case "performance":
{
newEntry.Logger = level;
newEntry.Priority = "INFO";
break;
}
}
}
}
Note that MyPostProcessor is a sample implementation of the ILogPostProcessor interface. This interface defines one method Modify(LogEntry entry).
public interface ILogPostProcessor
{
void Modify(LogEntry newEntry);
}
The Modify method can be used to modify the parsed log entry in any way you see fit. This method is called immediately after the entry is parsed, but before the log entry is given to LogViewPlus for display.
Post processors are reused across multiple new log entries. Therefore your custom post processor should not maintain any state.
Once we have compiled our custom post processor and added it into the LogViewPlus process (following the instructions outlined in running the samples), we still need to associate our post processor with a given log file. To do this, we can use the parser settings dialog. If LogViewPlus detects the possibility of a custom post processor, it will add a new field into the parser settings dialog. The new "post processor" field will allow you to associate a post processor with a parser and log file as shown below.
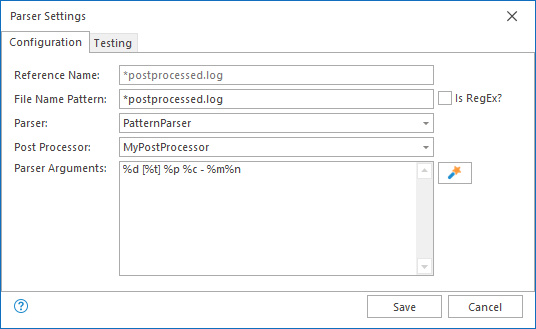
Once we have associated the post processor with a log file parser all we need to do is refresh the log file to see that our custom post processor is performing as expected.

As you can see, creating a custom post processor for LogViewPlus is relatively simple. If you have any questions or comments please feel free to contact us.